Gast Guest
 | Subject: Somethin to show Sun Mar 23, 2008 10:41 pm | |
| Yup, take a good look at the lottery. - Code:
-
using System; using System.Collections.Generic; using System.Text; using System.IO;
namespace CO_Full_Server { public class Lottery { /// <summary> /// Begins the lottery class. /// Copyright © 2007, Conquer Online 2.1 /// </summary> /// <param name="_Char"></param> public Lottery() {
} /// <summary> /// Generate the quality of item won. /// </summary> /// <param name="isFixed">Can item be 'Fixed'?</param> /// <returns>The quality of the item, from 3 - 9, or 0 if item can be 'Fixed'.</returns> int Quality(bool isFixed) { int probability = World.RND.Next(600); if (World.IsBetween(probability, 95, 100)) return 9; if (World.IsBetween(probability, 0, 30) && isFixed) return 0; return World.RND.Next(3, 8); }
/// <summary> /// Determines the additional HP added to the item. /// </summary> /// <returns>The Bonus HP, from 0 to 255.</returns> int Enchant() { int probability = World.RND.Next(200); if (World.IsBetween(probability, 50, 70)) return World.RND.Next(0, 50); else if (World.IsBetween(probability, 90, 110)) return World.RND.Next(50, 130); else if (World.IsBetween(probability, 130, 140)) return World.RND.Next(130, 256); return 0; }
/// <summary> /// Determines the bonus level of the item. /// </summary> /// <returns>The Bonus Level, from 0 to 9.</returns> int Plus() { int probability = World.RND.Next(200); if (World.IsBetween(probability, 50, 90)) return World.RND.Next(0, 4);//+0 - +3 else if (World.IsBetween(probability, 120, 160)) return World.RND.Next(4, 9); else if (World.IsBetween(probability, 95, 100)) return World.RND.Next(8, 10); return 0; }
/// <summary> /// Generates the Damage Subtraction percentage. /// </summary> /// <returns>A number between 1 to 7.</returns> int Bless() { int probability = World.RND.Next(350); if (World.IsBetween(probability, 50, 70)) return 1; else if (World.IsBetween(probability, 120, 160)) return 3; else if (World.IsBetween(probability, 200, 250)) return 6; else if (World.IsBetween(probability, 38, 68)) return 7; return 0; }
/// <summary> /// Generate the item ID of the item won from lottery. /// </summary> /// <returns>Returns the item ID of the item won.</returns> int Item() { if (World.DropFromFile) { restart: int intR = World.RND.Next(World.LottoItems.Count); string[] sItem = (string[])World.LottoItems[Convert.ToInt32(intR)]; if (sItem[1] == "1") return Convert.ToInt32(sItem[0]); int ItemID = Convert.ToInt32(sItem[0]); if (CanQual(ItemID)) { string strID = ItemID.ToString(); string strTmpID = strID.Remove(strID.Length - 1, 1) + "3"; int tmpID = Convert.ToInt32(strTmpID); string[] fullItem = World.DBItem(tmpID); if (fullItem[0] == "-1") goto restart; strTmpID = fullItem[0].Remove(fullItem[0].Length - 1, 1) + Quality(false).ToString(); tmpID = Convert.ToInt32(strTmpID); fullItem = World.DBItem(tmpID); if (fullItem[0] != "-1") return tmpID; goto restart; } else goto restart; } else { restart: int intR = World.RND.Next(BackendDB.ItemDatabase.Length); string[] sItem = (string[])BackendDB.ItemDatabase[Convert.ToInt32(intR)];
int ItemID = Convert.ToInt32(sItem[0]); if (CanQual(ItemID)) { string strID = ItemID.ToString(); string strTmpID = strID.Remove(strID.Length - 1, 1) + "3"; int tmpID = Convert.ToInt32(strTmpID); string[] fullItem = World.DBItem(tmpID); if (fullItem[0] == "-1") goto restart; strTmpID = fullItem[0].Remove(fullItem[0].Length - 1, 1) + Quality(false).ToString(); tmpID = Convert.ToInt32(strTmpID); fullItem = World.DBItem(tmpID); if (fullItem[0] != "-1") return tmpID; goto restart; } else if (World.DBItem(ItemID).Length != 1) return ItemID; else goto restart; } return 0; }
/// <summary> /// Determine if the item is equippable. /// </summary> /// <param name="ItemID">The ItemID of the item to check.</param> /// <returns>True if the item is equippable, and can have edits to its quality.</returns> bool CanQual(int ItemID) { if (World.IsBetween(ItemID, 111303, 182975)) return true; return false; }
/// <summary> /// Determine if the item can have a bonus level (Plus) added to it. /// </summary> /// <param name="ItemID">The ItemID of the item to check.</param> /// <returns>A boolean value. True if the item can be plussed. /// False if item cannot be plussed.</returns> bool CanPlus(int ItemID) { if (BackendDB.ItemPlus.Contains(Convert.ToInt32(ItemID.ToString().Remove(ItemID.ToString().Length - 1, 1) + "0"))) return true; return false; }
/// <summary> /// Return an integral array of the item won in the Lottery. /// </summary> /// <returns>A 12-value long array, containing all the data required.</returns> public int[] GetItem() { int[] FullItem = new int[12]; FullItem[0] = Item(); FullItem[3] = Plus(); FullItem[4] = Bless(); FullItem[5] = Enchant(); FullItem[9] = World.GenerateUID(); FullItem[7] = 50; FullItem[8] = 70; return FullItem; } } }
I made that for my own server, like? |
|
Guest Guest
 | Subject: Re: Somethin to show Sun Mar 23, 2008 10:44 pm | |
| hmmm dont know if it works but looks good  |
|
nearbosh Noob

Posts : 11 Join date : 2008-03-25 Age : 29 Location : Lost in thought
 | Subject: Re: Somethin to show Tue Mar 25, 2008 5:52 pm | |
| I'm guessing that's in C++? Gunna start learning C++ now. Only know a bit of java. | |
|
Demarquise Noob

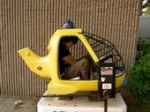
Posts : 9 Join date : 2008-04-12 Age : 31 Location : NC
 | Subject: Re: Somethin to show Wed Apr 16, 2008 1:50 pm | |
| I have no idea what this mean but I'll take it as a good thing >.>  | |
|
Buddie Bandit


Posts : 21 Join date : 2008-04-18 Age : 30 Location : USA, Oklahoma
 | Subject: Re: Somethin to show Fri Apr 18, 2008 10:33 pm | |
| yea idk wat it means either but good job?  | |
|
ihavenoname[AM] Admin

![ihavenoname[AM]](https://2img.net/u/1714/20/17/46/avatars/230-41.gif)
Posts : 63 Join date : 2008-04-15 Location : Miami
 | Subject: Re: Somethin to show Fri Apr 18, 2008 10:48 pm | |
| This topic's point has been made.
# locked | |
|
Sponsored content
 | Subject: Re: Somethin to show  | |
| |
|